Publish/Subscribe
12 Minute Read
This tutorial will introduce you to the fundamentals of the Solace Node.js API version 10 or later by connecting a client, adding a topic subscription and sending a message matching this topic subscription. This forms the basis for any publish / subscribe message exchange.
Assumptions
This tutorial assumes the following:
- You are familiar with Solace core concepts.
-
You have access to Solace messaging with the following configuration details:
- Connectivity information for a Solace message-VPN
- Enabled client username and password
One simple way to get access to Solace messaging quickly is to create a messaging service in Solace Cloud as outlined here. You can find other ways to get access to Solace messaging below.
Goals
The goal of this tutorial is to demonstrate the most basic messaging interaction using a Solace message router. This tutorial will show you:
- How to build and send a message on a topic
- How to subscribe to a topic and receive a message
Get Solace Messaging
This tutorial requires access Solace PubSub+ messaging and requires that you know several connectivity properties about your Solace messaging. Specifically you need to know the following:
Resources | Value | Description |
---|---|---|
Host | String | This is the address clients use when connecting to the PubSub+ messaging to send and receive messages. (Format: DNS_NAME:Port or IP:Port ) |
Message VPN | String | The PubSub+ message router Message VPN that this client should connect to. |
Client Username | String | The client username. (See Notes below) |
Client Password | String | The client password. (See Notes below) |
There are several ways you can get access to PubSub+ Messaging and find these required properties.
Option 1: Use PubSub+ Cloud
- Follow these instructions to quickly spin up a cloud-based PubSub+ messaging service for your applications.
-
The messaging connectivity information is found in the service details in the connectivity tab (shown below). You will need:
- Host:Port (use the SMF URI)
- Message VPN
- Client Username
- Client Password
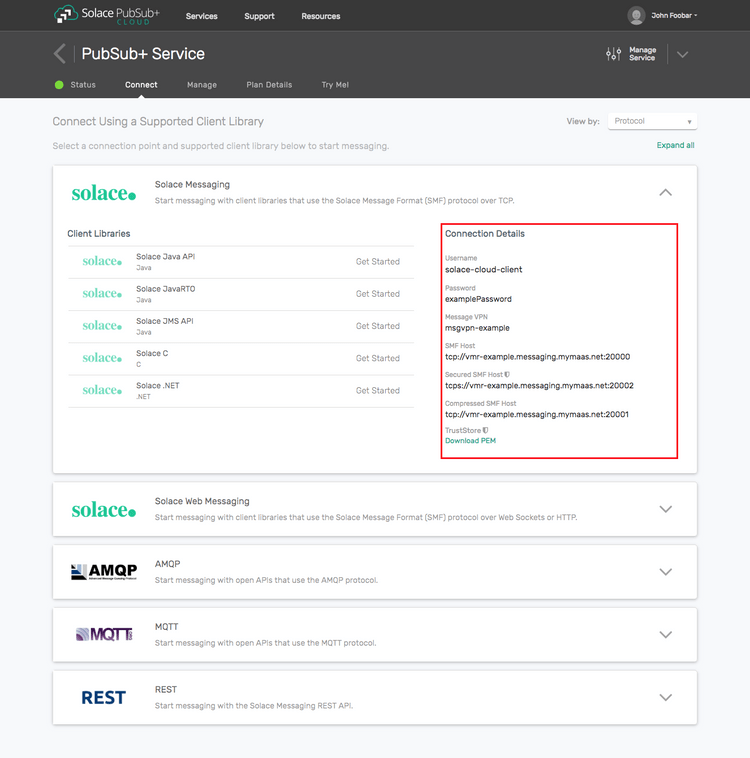
Option 2: Start a PubSub+ Software
-
Follow these instructions to start the PubSub+ Software in leading Clouds, Container Platforms or Hypervisors. The tutorials outline where to download and how to install the PubSub+ Software.
-
The messaging connectivity information are the following:
-
Host: <public_ip> (IP address assigned to the VMR in tutorial instructions)
-
Message VPN: default
-
Client Username: sampleUser (can be any value)
-
Client Password: samplePassword (can be any value)
Note: By default, the PubSub+ Software "default" message VPN has authentication disabled.
-
Option 3: Get access to a PubSub+ Appliance
-
Contact your PubSub+ appliance administrators and obtain the following:
- A PubSub+ Message-VPN where you can produce and consume direct and persistent messages
- The host name or IP address of the Solace appliance hosting your Message-VPN
- A username and password to access the Solace appliance
Obtaining the Solace PubSub+ API
The repository where this tutorial reside already comes with C API library version 7.7.1.4. However, you should always check for any newer version for download here. The C API is distributed as a gzipped tar file for all supported platform. To update to a newer version of the API, please ensure that the existing core library components are appropriately replaced by the newer components.
Loading and Initializing the Solace JavaScript API
To load the Solace Web Messaging API for JavaScript on your HTML page simply include the solclient.js
file from the distribution.
<head>
<script src="../../../lib/solclient.js"></script>
</head>
Use the debug version of the API in lib/solclient-debug.js
file instead, if you’re planning to see console log messages and/or debug it.
<head>
<script src="../../../lib/solclient-debug.js"></script>
</head>
Then initialize the SolclientFactory
, which is the first entry point to the API. Add the following to initialize with the latest version10
behavior profile to run with the default property values that Solace recommends at the time of the version 10 release.
var factoryProps = new solace.SolclientFactoryProperties();
factoryProps.profile = solace.SolclientFactoryProfiles.version10;
solace.SolclientFactory.init(factoryProps);
If the debug version of the API has been loaded the required level of logging can be set like so:
solace.SolclientFactory.setLogLevel(solace.LogLevel.DEBUG);
Connecting to the Solace message router
In order to send or receive messages, an application must connect a Solace session. The Solace session is the basis for all client communication with the Solace message router.
The solace.SolclientFactory
is used to create a Solace Session
from SessionProperties
. In the example below, SessionProperties
is created using object initializers.
Then listeners are defined for Session Events of interest and for receiving direct messages, which are explained in the next sections.
The created session connects to the Solace message router with the session.connect()
call.
This tutorial’s sample code comes as two separate applications: one (with the "publisher" object) publishes messages to a specific topic, and the other (with the "subscriber" object) subscribes to messages on that topic and receives the messages.
The following is an example of a session creating and connecting to the Solace message router for the subscriber. The publisher's code will be the same except for that it doesn't require a message event listener.
// create session
subscriber.session = solace.SolclientFactory.createSession({
// solace.SessionProperties
url: hosturl,
vpnName: vpn,
userName: username,
password: pass,
});
// define session event listeners
/*...see section Session Events...*/
// define message event listener
/*...see section Receiving a message...*/
// connect the session
try {
subscriber.session.connect();
} catch (error) {
subscriber.log(error.toString());
}
At this point your Node.js application is connected as a client to the Solace message router. You can use PubSub+ Manager to view this client connection and related details.
Session Events
The Solace Node.js API communicates changes in status and results of connect and subscription calls through emitting session events with certain event names.
It is necessary to wire your application logic to events through listeners to take appropriate action. The most important events are:
SessionEventCode.UP_NOTICE
: success connecting session to the Solace message routerSessionEventCode.CONNECT_FAILED_ERROR
: unable to connect to the Solace message routerSessionEventCode.DISCONNECTED
: session was disconnected from the Solace message routerSessionEventCode.SUBSCRIPTION_OK
: subscription to a topic was successfully created on the Solace message router
This is how event listeners can be defined in the sample publisher:
// define session event listeners
publisher.session.on(solace.SessionEventCode.UP_NOTICE, function (sessionEvent) {
publisher.log('=== Successfully connected and ready to publish messages. ===');
publisher.publish();
publisher.exit();
});
publisher.session.on(solace.SessionEventCode.CONNECT_FAILED_ERROR, function (sessionEvent) {
publisher.log('Connection failed to the message router: ' + sessionEvent.infoStr +
' - check correct parameter values and connectivity!');
});
publisher.session.on(solace.SessionEventCode.DISCONNECTED, function (sessionEvent) {
publisher.log('Disconnected.');
if (publisher.session !== null) {
publisher.session.dispose();
publisher.session = null;
}
});
Note that the application logic can be triggered only after receiving the solace.SessionEventCode.UP_NOTICE
event:
publisher.session.on(solace.SessionEventCode.UP_NOTICE, function (sessionEvent) {
publisher.log('=== Successfully connected and ready to publish messages. ===');
publisher.publish();
publisher.exit();
});
On the subscriber side we also might want to implement reaction to subscription error and to subscription added or removed:
// define session event listeners
/*...SNIP...*/
subscriber.session.on(solace.SessionEventCode.SUBSCRIPTION_ERROR, function (sessionEvent) {
subscriber.log('Cannot subscribe to topic: ' + sessionEvent.correlationKey);
});
subscriber.session.on(solace.SessionEventCode.SUBSCRIPTION_OK, function (sessionEvent) {
if (subscriber.subscribed) {
subscriber.subscribed = false;
subscriber.log('Successfully unsubscribed from topic: ' + sessionEvent.correlationKey);
} else {
subscriber.subscribed = true;
subscriber.log('Successfully subscribed to topic: ' + sessionEvent.correlationKey);
subscriber.log('=== Ready to receive messages. ===');
}
});
The subscriber application logic is also triggered only after receiving the solace.SessionEventCode.UP_NOTICE
event:
subscriber.session.on(solace.SessionEventCode.UP_NOTICE, function (sessionEvent) {
subscriber.log('=== Successfully connected and ready to subscribe. ===');
subscriber.subscribe();
});
See solace.SessionEventCode
in the Node.js API Reference for the full list of session event codes.
Receiving a message
This tutorial uses “Direct” messages which are at most once delivery messages. So first, let’s create a listener and express interest in the messages by subscribing to a Solace topic. Then you can look at publishing a matching message and see it received.
With a subscriber session created in the previous step, we declare a message event listener.
// define session event listeners
/*...see section Session Events...*/
// define message event listener
subscriber.session.on(solace.SessionEventCode.MESSAGE, function (message) {
subscriber.log('Received message: "' + message.getBinaryAttachment() + '", details:\n' + message.dump());
'", details:\n' + message.dump());
});
// connect the session
When a message is received, this listener is called with the message as parameter.
You must subscribe to a topic in order to express interest in receiving messages. This tutorial uses the topic “tutorial/topic”.
subscriber.subscribe = function () {
/*...SNIP...*/
try {
subscriber.session.subscribe(
solace.SolclientFactory.createTopic("tutorial/topic"),
true,
"tutorial/topic",
10000
);
} catch (error) {
subscriber.log(error.toString());
}
/*...SNIP...*/
}
Notice parameters to the session subscribe
function.
- The first parameter is the subscription topic.
- The second (boolean) parameter specifies whether a corresponding event will be generated when the subscription is added successfully.
- The third parameter is the correlation key. This parameters value will be returned to the
SUBSCRIPTION_OK
session event listener for as thecorrelationKey
property of the event:event.correlationKey
. - The fourth parameter is the function call timeout. The timeout sets the limit in milliseconds the
subscribe
function is allowed to block the execution thread. If this limit is reached and the subscription is still not added, then an exception is thrown.
After the subscription is successfully added the subscriber is ready to receive messages and nothing happens until a message is received.
Sending a message
Now it is time to send a message to the waiting consumer.
Creating and sending the message
To send a message to a topic, you must create a message and a topic type destination. Both of these are created from the solace.SolclientFactory
.
This tutorial uses “Direct” messages which are at most once delivery messages and will send a message with Text contents “Sample Message”.
This is how it is done in the sample publisher code:
var messageText = 'Sample Message';
var message = solace.SolclientFactory.createMessage();
message.setDestination(solace.SolclientFactory.createTopicDestination(publisher.topicName));
message.setBinaryAttachment(messageText);
message.setDeliveryMode(solace.MessageDeliveryModeType.DIRECT);
if (publisher.session !== null) {
try {
publisher.session.send(message);
publisher.log('Message published.');
} catch (error) {
publisher.log(error.toString());
}
} else {
publisher.log('Cannot publish because not connected to Solace message router.');
}
At this point a message to the Solace message router has been sent and your waiting consumer will have received the message and printed its contents to the Node.js console.
Summarizing
Combining the example source code shown above results in the following source code files:
Getting the Source
Clone the GitHub repository containing the Solace samples.
git clone https://github.com/SolaceSamples/solace-samples-nodejs
cd solace-samples-nodejs
Note: the code in the master
branch of this repository depends on Solace Node.js API version 10 or later. If you want to work with an older version clone the branch that corresponds your version.
Installing the Node.js API
For a local installation of the API package, run from the current solace-samples-nodejs
directory:
npm install
Running the Samples
The samples consist of two separate publisher and subscriber Node.js applications in the /src/basic-samples
directory: TopicPublisher.js
and TopicSubsciber.js
.
The publisher application publishes one message and exits, the subscriber application is running until Ctrl-C is pressed on the console.
Sample Output
First run TopicSubscriber.js
in Node.js, giving it following arguments:
node TopicSubscriber.js <protocol://host:port> <client-username>@<message-vpn> <client-password>
The following is the output of the tutorial’s TopicSubscriber.js
application after it successfully connected to the Solace message router and subscribed to the subscription topic.
$ node TopicSubscriber.js ws://192.168.133.64 testuser@default passw
[15:38:11]
*** Subscriber to topic "tutorial/topic" is ready to connect ***
[15:38:11] Connecting to Solace message router using url: ws://192.168.133.64
[15:38:11] Client username: testuser
[15:38:11] Solace message router VPN name: default
[15:38:11] Press Ctrl-C to exit
[15:38:11] === Successfully connected and ready to subscribe. ===
[15:38:11] Subscribing to topic: tutorial/topic
[15:38:11] Successfully subscribed to topic: tutorial/topic
[15:38:11] === Ready to receive messages. ===
Now, run TopicPublisher.js
in Node.js, also specifying the same arguments.
node TopicPublisher.js <protocol://host:port> <client-username>@<message-vpn> <client-password>
It will connect to the router, publish a message and exit.
The following is the output of the tutorial’s TopicPublisher.js
application after it successfully connected to the Solace message router, published a message and exited.
$ node TopicPublisher.js ws://192.168.133.64 testuser@default passw
[15:38:18]
*** Publisher to topic "tutorial/topic" is ready to connect ***
[15:38:18] Connecting to Solace message router using url: ws://192.168.133.64
[15:38:18] Client username: testuser
[15:38:18] Solace message router VPN name: default
[15:38:19] === Successfully connected and ready to publish messages. ===
[15:38:19] Publishing message "Sample Message" to topic "tutorial/topic"...
[15:38:19] Message published.
[15:38:19] Disconnecting from Solace message router...
[15:38:19] Disconnected.
This is the subscriber receiving a message (TopicSubscriber.js)
:
[15:38:19] Received message: "Sample Message", details:
Destination: [Topic tutorial/topic]
Class Of Service: COS1
DeliveryMode: DIRECT
Expiration: 0 (Wed Dec 31 1969 19:00:00 GMT-0500 (Eastern Standard Time))
Binary Attachment: len=14
53 61 6d 70 6c 65 20 4d 65 73 73 61 67 65 Sample.Message