Publish/Subscribe
8 Minute Read
This tutorial will introduce you to the fundamentals of the Solace PubSub+ API by connecting a client, adding a topic subscription and sending a message matching this topic subscription. This forms the basis for any publish / subscribe message exchange.
Assumptions
This tutorial assumes the following:
- You are familiar with Solace PubSub+ core concepts.
- You have access to PubSub+ messaging with the following configuration details:
- Connectivity information for a PubSub+ message-VPN
- Enabled client username and password
One simple way to get access to PubSub+ messaging quickly is to create a messaging service in PubSub+ Cloud as outlined here. You can find other ways to get access to PubSub+ messaging below.
Goals
The goal of this tutorial is to demonstrate the most basic messaging interaction using Solace PubSub+. This tutorial will show you:
- How to build and send a message on a topic
- How to subscribe to a topic and receive a message
Get Solace Messaging
This tutorial requires access Solace PubSub+ messaging and requires that you know several connectivity properties about your Solace messaging. Specifically you need to know the following:
Resources | Value | Description |
---|---|---|
Host | String | This is the address clients use when connecting to the PubSub+ messaging to send and receive messages. (Format: DNS_NAME:Port or IP:Port ) |
Message VPN | String | The PubSub+ message router Message VPN that this client should connect to. |
Client Username | String | The client username. (See Notes below) |
Client Password | String | The client password. (See Notes below) |
There are several ways you can get access to PubSub+ Messaging and find these required properties.
Option 1: Use PubSub+ Cloud
-
Follow these instructions to quickly spin up a cloud-based PubSub+ messaging service for your applications.
-
The messaging connectivity information is found in the service details in the connectivity tab (shown below). You will need:
- Host:Port (use the SMF URI)
- Message VPN
- Client Username
- Client Password
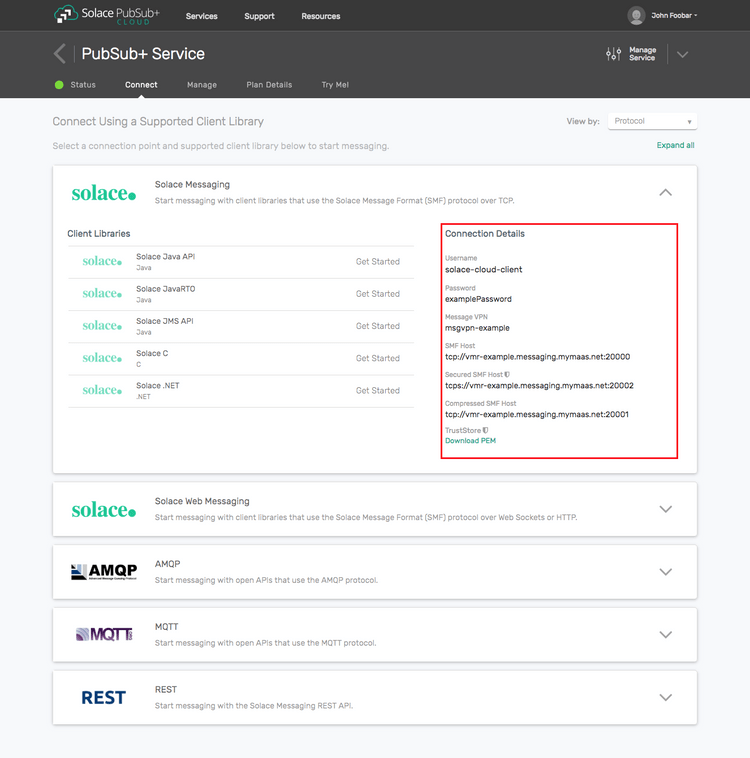
Option 2: Start a PubSub+ Software
-
Follow these instructions to start the PubSub+ Software in leading Clouds, Container Platforms or Hypervisors. The tutorials outline where to download and how to install the PubSub+ Software.
-
The messaging connectivity information are the following:
-
Host: <public_ip> (IP address assigned to the VMR in tutorial instructions)
-
Message VPN: default
-
Client Username: sampleUser (can be any value)
-
Client Password: samplePassword (can be any value)
Note: By default, the PubSub+ Software "default" message VPN has authentication disabled.
-
Option 3: Get access to a PubSub+ Appliance
-
Contact your PubSub+ appliance administrators and obtain the following:
- A PubSub+ Message-VPN where you can produce and consume direct and persistent messages
- The host name or IP address of the Solace appliance hosting your Message-VPN
- A username and password to access the Solace appliance
Obtaining the Solace PubSub+ API
The latest versions of all Solace APIs can be downloaded from the solace downloads page. Otherwise, check the public samples repository of the API where the library is already packaged as a gzipped tar file. Note: always check for the latest API version!
Connecting to the Solace PubSub+ message router
In order to send or receive messages, an application must connect a PubSub+ session. The PubSub+ session is the basis for all client communication with the PubSub+ message router.
In the Solace messaging API for C (SolClient), a few distinct steps are required to create and connect a Solace session.
- The API must be initialized
- Appropriate asynchronous callbacks must be declared
- A SolClient Context is needed to control application threading
- The SolClient session must be created
Initializing the CCSMP API
To initialize the SolClient API, you call the initialize method with arguments that control logging.
/* solClient needs to be initialized before any other API calls. */
solClient_initialize ( SOLCLIENT_LOG_DEFAULT_FILTER, NULL );
This call must be made prior to making any other calls to the SolClient API. It allows the API to initialize internal state and buffer pools.
SolClient Asynchronous Callbacks
The SolClient API is predominantly an asynchronous API designed for the highest speed and lowest latency. As such most events and notifications occur through callbacks. In order to get up and running, the following basic callbacks are required at a minimum.
static int msgCount = 0;
solClient_rxMsgCallback_returnCode_t
sessionMessageReceiveCallback ( solClient_opaqueSession_pt opaqueSession_p, solClient_opaqueMsg_pt msg_p, void *user_p )
{
printf ( "Received message:\n" );
solClient_msg_dump ( msg_p, NULL, 0 );
printf ( "\n" );
msgCount++;
return SOLCLIENT_CALLBACK_OK;
}
void
sessionEventCallback ( solClient_opaqueSession_pt opaqueSession_p,
solClient_session_eventCallbackInfo_pt eventInfo_p, void *user_p )
{
printf("Session EventCallback() called: %s\n", solClient_session_eventToString ( eventInfo_p->sessionEvent));
}
The messageReceiveCallback is invoked for each message received by the Session. In this sample, the message is printed to the screen.
The eventCallback is invoked for various significant session events like connection, disconnection, and other SolClient session events. In this sample, simply prints the events. See the SolClient API documentation and samples for details on the session events.
Context Creation
As outlined in the core concepts, the context object is used to control threading that drives network I/O and message delivery and acts as containers for sessions. The easiest way to create a context is to use the context initializer with default thread creation.
/* Context */
solClient_opaqueContext_pt context_p;
solClient_context_createFuncInfo_t contextFuncInfo = SOLCLIENT_CONTEXT_CREATEFUNC_INITIALIZER;
solClient_context_create ( SOLCLIENT_CONTEXT_PROPS_DEFAULT_WITH_CREATE_THREAD,
&context_p, &contextFuncInfo, sizeof ( contextFuncInfo ) );
Session Creation
Finally a session is needed to actually connect to the PubSub+ message router. This is accomplished by creating a properties array and connecting the session.
/* Session */
solClient_opaqueSession_pt session_p;
solClient_session_createFuncInfo_t sessionFuncInfo = SOLCLIENT_SESSION_CREATEFUNC_INITIALIZER;
/* Session Properties */
const char *sessionProps[50] = {0, };
int propIndex = 0;
char *username,*password,*vpnname,*host;
/* Configure the Session function information. */
sessionFuncInfo.rxMsgInfo.callback_p = sessionMessageReceiveCallback;
sessionFuncInfo.rxMsgInfo.user_p = NULL;
sessionFuncInfo.eventInfo.callback_p = sessionEventCallback;
sessionFuncInfo.eventInfo.user_p = NULL;
/* Configure the Session properties. */
propIndex = 0;
host = argv[1];
vpnname = argv[2];
username = strsep(&vpnname,"@");
password = argv[3];
sessionProps[propIndex++] = SOLCLIENT_SESSION_PROP_HOST;
sessionProps[propIndex++] = host;
sessionProps[propIndex++] = SOLCLIENT_SESSION_PROP_VPN_NAME;
sessionProps[propIndex++] = vpnname;
sessionProps[propIndex++] = SOLCLIENT_SESSION_PROP_USERNAME;
sessionProps[propIndex++] = username;
sessionProps[propIndex++] = SOLCLIENT_SESSION_PROP_PASSWORD;
sessionProps[propIndex++] = password;
sessionProps[propIndex++] = NULL;
/* Create the Session. */
solClient_session_create ( ( char ** ) sessionProps,
context_p,
&session_p, &sessionFuncInfo, sizeof ( sessionFuncInfo ) );
/* Connect the Session. */
solClient_session_connect ( session_p );
printf ( "Connected.\n" );
When creating the session, the factory method takes the session properties, the session pointer and information about the session callback functions. The API then creates the session within the supplied context and returns a reference in the session pointer. The final call to solClient_session_connect establishes the connection to the PubSub+ message router which makes the session ready for use.
At this point your client is connected to the PubSub+ message router. You can use PubSub+ Manager to view the client connection and related details.
Receiving a message
This tutorial is uses "Direct" messages which are at most once delivery messages. So first, let's express interest in the messages by subscribing to a PubSub+ topic. Then you can look at publishing a matching message and see it received.
With a session connected in the previous step, then you must subscribe to a topic in order to express interest in receiving messages. This tutorial uses the topics "topic/topic1".
solClient_session_topicSubscribeExt ( session_p,
SOLCLIENT_SUBSCRIBE_FLAGS_WAITFORCONFIRM,
"topic/topic1");
Then after the subscription is added, the consumer is started. At this point the consumer is ready to receive messages. So wait in a loop for the expected message to arrive.
printf ( "Waiting for message......\n" );
fflush ( stdout );
while ( msgCount < 1 ) {
sleepInSec ( 1 );
}
Sending a message
Now it is time to send a message to the waiting consumer.
To send a message, you must create a message and a topic destination. This tutorial will send a PubSub+ binary message with contents "Hello world!". Then you must send the message to the PubSub+ message router.
/* Message */
solClient_opaqueMsg_pt msg_p = NULL;ss
solClient_destination_t destination;
const char *text_p = "Hello world!";
/* Allocate memory for the message that is to be sent. */
solClient_msg_alloc ( &msg_p );
/* Set the message delivery mode. */
solClient_msg_setDeliveryMode ( msg_p, SOLCLIENT_DELIVERY_MODE_DIRECT );
/* Set the destination. */
destination.destType = SOLCLIENT_TOPIC_DESTINATION;
destination.dest = "tutorial/topic";
solClient_msg_setDestination ( msg_p, &destination, sizeof ( destination ) );
/* Add some content to the message. */
solClient_msg_setBinaryAttachment ( msg_p, text_p, ( solClient_uint32_t ) strlen ( (char *)text_p ) );
/* Send the message. */
printf ( "About to send message '%s' to topic '%s'...\n", (char *)text_p, argv[4] );
solClient_session_sendMsg ( session_p, msg_p );
printf ( "Message sent. Exiting.\n" );
solClient_msg_free ( &msg_p );
In the SolClient API, messages are allocated and freed from an internal API message pool for greatest performance and efficiency. Therefore as shown, messages must be acquired by calls to solClient_msg_alloc and then later freed back to the pool by calls to solClient_msg_free.
The minimum properties required to create a SolClient message that can be sent is to set the delivery mode, queue or topic destination, and message contents as shown in the above code. Once the message is created it is sent to the PubSub+ message router with a call to solClient_session_sendMsg.
At this point the producer has sent a message to the PubSub+ message router and your waiting consumer will have received the message and printed its contents to the screen.
Summarizing
The full source code for this example is available in GitHub. If you combine the example source code shown above results in the following source:
The OS source code simply provides platform abstraction. The subscriber sample makes use of this for the sleep in the main loop.
Building
Building these examples is simple.
For linux and mac
All you need is to execute the compile script in the build
folder.
linux
build$ ./build_intro_linux_xxx.sh
mac
build$ ./build_intro_mac_xxx.sh
For windows
You can either build the examples from DOS prompt or from Visual Studio IDE.
To build from DOS prompt, you must first launch the appropriate Visual Studio Command Prompt and then run the batch file
c:\solace-sample-c\build>build_intro_win_xxx.bat
Referencing the downloaded SolClient library include and lib file is required. For more advanced build control, consider adapting the makefile found in the "intro" directory of the SolClient package. The above samples very closely mirror the samples found there.
Running the Samples
If you start the TopicSubscriber
with the required arguments of your PubSub+ messaging, it will connect and wait for a message.
bin$ . ./setenv.sh
bin$ ./TopicSubscriber <msg_backbone_ip:port> <message-vpn> <client-username> <password> <topic>
TopicSubscriber initializing...
Connected.
Waiting for message......
Then you can send a message using the TopicPublisher
with the same arguments. If successful, the output for the producer will look like the following:
bin$ ./TopicPublisher <msg_backbone_ip:port> <message-vpn> <client-username> <password> <topic>
TopicSubscriber initializing...
Connected.
About to send message 'Hello world!' to topic 'topic/topic1'...
Message sent. Exiting.
With the message delivered the subscriber output will look like the following:
Received message:
Destination: Topic 'topic/topic1'
Class Of Service: COS_1
DeliveryMode: DIRECT
Binary Attachment: len=12
48 65 6c 6c 6f 20 77 6f 72 6c 64 21 Hello wo rld!
Exiting.
The received message is printed to the screen. The message contents was "Hello world!" as expected and shown in the contents of the message dump along with additional information about the PubSub+ message that was received.
You have now successfully connected a client, subscribed to a topic and exchanged messages using this topic.